Comparison Operators
== if equal
! not
!= if not equal
< if less
<= less than or equal to
> if large
>= greater than or equal to
Logical Operators
Sometimes we may have multiple conditions that need to be checked. In this case the following two operators are used.
And Operator ( && ):
The condition is considered fulfilled when all of the specified conditions are true. If even one of the conditions is false, the condition is deemed not met.
Example: The program that will accept the number entered in TextBox1 when it is greater than 0 and less than 100, otherwise it will throw an error:
int not1 = int.Parse(textBox1.Text);
if (note1 >= 0 && not1 <= 100)
{
MessageBox.Show("Information entered");
}
else
{
MessageBox.Show("You entered incorrectly.");
}
Or Operator ( || ):
If one of the specified conditions is true, it is sufficient for the condition to be considered true. If all of the conditions are false, the condition is deemed not met.
Example: If the age entered in the box is greater than 65 or checkbox1 is checked, the program that gives a message "Free", otherwise "25 TL":
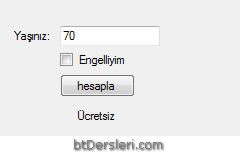
int age = int.Parse(textBox1.Text);
if (age >= 65 || checkBox1.Checked == true)
{
MessageBox.Show("Free");
}
else
{
MessageBox.Show("25 TL");
}
Logical Operation Examples
Some variables are defined and value given below. Next, let's examine the results of the logical tests.
int x = 5, y = 15, z = 3, number1 = 5;
string name = "Sumer";
bool k;
k = x > y; // the result is k = false.
k = x < y && x > z; // the result is k = true.
k = (x == y || x == z || x == number1 ); // the result is k = true.
k = (x +10 >= y || x > number1 ) && ( 23 > number1 && 5 == 5 ); // the result is k = false.
visual c# comparison operators and logical operators, comparison in c#, logical operations in c#
EXERCISES
Logical operation tutorials in c#
|
|
-
Program to find out if the entered number is odd or even
-
If the entered number is divisible by both 2 and 3, “OK”, otherwise “ERROR” message.
-
"Multiple of 2 and 3" if the entered number is divisible by both 2 and 3, "multiple of 2" if it is only divisible by 2, "multiple of 3" if it is only divisible by 3, neither 2 nor 3' If it is not divisible by e, the program that gives the message "not a multiple of 2 or 3"
-
If the entered number is divisible by both 4, 5, and 6, the program displays the message "Number OK", otherwise "Number Not Available".
-
If the entered number is divisible by at least one of the numbers 4, 5 and 6, the program that gives the message "Number OK", otherwise "Number Not Available".
-
If the entered number is between 0 and 100 and is even, the program displays "Number OK", otherwise "Number Not Available".
-
If at least one of the 3 numbers entered is greater than 50, the program gives the message "Sufficient", otherwise "Insufficient"
-
If the 3 numbers entered are all greater than 0 and even, the program displays “Successful Login”, otherwise “Failed login”.
Read 1278 times.