if - else if Control Structure
In our previous lesson, we explained the use of the if else structure.
if (our condition)
{
// Operations if true (if block)
}
else
{
//Operations if false (else block)
}
With the above structure, we can check a single condition and take different actions depending on whether it is correct or not.
However, if the number of cases that we need to check in our program is more than 2, we can specify what to do in each case separately by using else if .
For example, if we want to convert the student grade over 100 to the 5th system, we can use the else if structure as follows:
if ( your grade < 0)
{
label1.Text = "Bad Entry!";
}
else if (your grade < 50)
{
label1.Text = "1";
}
else if (your grade < 60)
{
label1.Text = "2";
}
else if (your grade < 70)
{
label1.Text = "3";
}
else if (your grade < 85)
{
label1.Text = "4";
}
else if (your grade <= 100)
{
label1.Text = "5";
}
else
{
label1.Text = "Bad Entry!";
}
We should consider the working logic of these codes as follows. The program will start to run and first encounter the if line and the condition here. If this condition is true, the if block will be made and other conditions and blocks will not be looked at.
If this condition is not met, the else if blocks will be checked in order and if there is a fulfilled condition, the transactions in that block will be performed.
In other words, the block of whichever condition is first fulfilled while descending from the top will work, and the next ones will be skipped regardless.
Else part is still optional and in case none of the above conditions are met, the transactions written here are done.
According to this logic, let's imagine the running moment of the above program as follows. User enter 55 as note information. In this case, the first two conditions do not hold. The following conditions hold. Because the number 55 complies with the condition of being less than 60, but it is also less than 70, 85 and 100.
In this case, will each of the blocks belonging to these conditions be processed?
Answer is no! Since the first condition to be fulfilled will be the condition in the 3rd order, 3 will be written in label1 and other blocks will be skipped.
c# else if tutorials, if else examples, else examples, if else usage
EXERCISES
Generating Random Number with As Many Digits as Selected
|
|
There are 1, 2, 3, 4 options in a ComboBox. Here, a random number will be generated with as many digits as the selected number and the result will be written to label1. For example, if 2 is selected from the ComboBox, a number between 10 and 99 will be generated, and when 3 is selected, a number between 100 and 999 will be generated.
Random nesne1 = new Random();
if (comboBox1.Text == "1")
label1.Text = (nesne1.Next(0, 9)).ToString();
else if (comboBox1.Text == "2")
label1.Text = (nesne1.Next(10, 99)).ToString();
else if (comboBox1.Text == "3")
label1.Text = (nesne1.Next(100, 999)).ToString();
else if (comboBox1.Text == "4")
label1.Text = (nesne1.Next(1000, 9999)).ToString();
Write the program that adds the relevant counties to the Listbox according to the selection made from the ComboBox object.
When the selection is made, all elements of the listBox should be cleared with the Clear method. Then, the districts should be added one by one with the Add method.
From the numbers entered in the two text boxes, the first number will be divided by the second and the result will be written to label1. If one of the boxes is empty or the second number 0 is entered, an error should be given.
if (textBox1.Text == "" || textBox2.Text == "" || textBox2.Text=="0")
label1.Text = "Check the entered information.";
else
{
double a = double.Parse(textBox1.Text);
double b = double.Parse(textBox2.Text);
double c = a / b;
label1.Text = c.ToString();
}
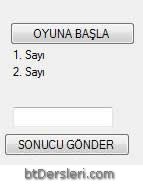
A game program will be written that attempts to teach addition.
When the Start Game button is clicked, the computer will generate 2 random numbers (between 0 and 100) and write these numbers into the labels that appear on the screen.
The user will calculate the sum of these two numbers head-on and enter the result in the text box on the right and press the send result button.
When the "Send Result" button is pressed, the value entered by the user will be checked and if it is equal to the sum of the two numbers that have just been generated, a message will be displayed as "CONGRATULATIONS", otherwise "ERROR".
A taxi parked in a car park costs 5TL for 1 hour, 10TL for 1 hour for a minibus, and 15TL for 1 hour for a commercial vehicle. In addition, for each hour after the first 1 hour, an additional 50% must be paid. Accordingly, after entering the vehicle type and the time left on the keyboard, write the codes of the program that writes the parking fee to be paid on the screen.
(For example, if a taxi stays for 3 hours, they will pay 5 TL for the first hour and 2.5 TL for the other hours (50% of them will pay 2.5 TL), and a total of 10 TL will be paid.)
Write the program that asks the user to enter a number between 0 and 1000 and writes it to the label as 4 digits by adding 0 or 0s in front of the entered number.
For example, if the user enters 521, it will write 0521 in label1, and if the user enters 63, it will write 0063.
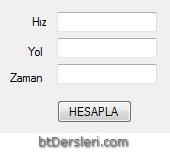
Make a program that will write the answer in that box by calculating which box is left blank when the button is pressed, using the formula Speed=Distance / time. You can even print the answer in red in the blank box.
(Of course, the user has to fill in two boxes.)
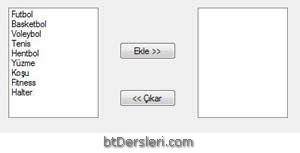
In a form like the one above, two listboxes and two buttons will be used. When the Add button is clicked, the selected item from the left list will be added to the right list and deleted from the left one.
Likewise, when a selection is made from the list on the right and the remove button is pressed, this time it will be removed from the list and added to the one on the left.
(warn the user when the button is pressed without making a selection (if(listbox1.selectedIndex==-1))
The user will enter their first and last name in the boxes and choose their gender. When you press the button, a message like this will be written in label1:
Welcome, Miss Elizabeth Hurley.
Read 1116 times.